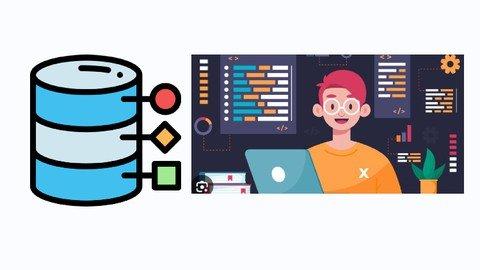
Published 5/2023
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz
Language: English | Size: 2.87 GB | Duration: 11h 9m
Mastering Data and Code: Unleashing the Power of PostgreSQL and Python to Build Dynamic Apps and APIs
What you'll learn
Installing and configuring PostgreSQL.
Understanding the fundamental concepts of PostgreSQL databases.
Creating PostgreSQL databases and tables.
Understanding and utilizing PostgreSQL data types.
Understanding the concept of CRUD (Create, Read, Update, Delete) operations.
Performing CRUD operations in PostgreSQL.
Understanding and utilizing table joins in PostgreSQL.
Performing various types of PostgreSQL table joins like Inner Join, Left Join, Right Join, and Full Outer Join.
Understanding and utilizing PostgreSQL aggregate functions like COUNT, SUM, AVG, MAX, and MIN.
Creating and using PostgreSQL triggers for automatic database operations.
Basic Python programming syntax and concepts.
Understanding and using Python variables and data types.
Implementing conditional statements in Python.
Understanding and utilizing loops in Python.
Writing and calling Python functions.
Using Jupyter Notebook for Python coding and testing.
Connecting a PostgreSQL database with a Python application.
Performing database operations in Python using PostgreSQL.
Understanding the structure of a database-driven Python application.
Designing and building a database-driven Python application.
Introduction to API and their importance.
Basics of the Django framework and Django REST Framework.
Building APIs using Django and Django REST Framework.
Connecting an API to a PostgreSQL database.
Making API calls from a Python application.
Understanding the principles of the REST architecture.
Testing and debugging a Python application connected to a PostgreSQL database.
Requirements
Basic Computing Skills: Students should be comfortable with using a computer, installing software, and navigating the internet.
Familiarity with Programming: While not absolutely necessary, some familiarity with programming concepts would be advantageous. This could be in any language, not necessarily Python or SQL.
Understanding of Databases: Basic understanding of what a database is and why it is used can be helpful. Familiarity with SQL would be a bonus but is not required.
Python Installation: It's recommended to have a recent version of Python (3.x) installed on your system. You should also install Jupyter Notebook, which is a popular tool for running Python code. The installations are covered in the course.
PostgreSQL Installation: You'll need to install PostgreSQL on your system. Don't worry if you're not sure how to do this, as the course will guide you through the process.
Willingness to Learn: Most importantly, you should be curious and willing to learn new concepts and tools. This is a hands-on course, so you'll need to be ready to get involved and experiment with the code and concepts.
While meeting all these prerequisites will help you get the most out of the course, don't be discouraged if you don't meet them all right now. The course is designed to be accessible even to beginners, and we'll be covering all necessary foundational concepts as we go. The most important requirement is a willingness to learn.
Description
Dive deep into the world of Python programming and PostgreSQL databases with our comprehensive course designed to take you from beginner to expert. In this dynamic and engaging course, you will learn the essential concepts and practices used by professional programmers and database administrators worldwide.This course begins by guiding you through the PostgreSQL Setup, introducing you to the world of relational databases. You will gain a solid understanding of PostgreSQL Database Fundamentals, including database schemas, data types, and relationships.Once you're comfortable with the basics, we'll venture into Performing PostgreSQL CRUD Operations, where you'll learn how to Create, Read, Update, and Delete data in your PostgreSQL databases. You'll then discover the power of PostgreSQL Table Joins, which allow you to combine data from multiple tables in powerful and insightful ways.We'll also delve into advanced SQL concepts, including PostgreSQL Aggregate Functions for summarizing data, and PostgreSQL Triggers which allow automatic actions based on database events.Shifting gears, we'll introduce you to Python, one of the world's most popular and powerful programming languages. You will get hands-on experience with Performing basic Python operations like Loops, conditional statements, Variables, and functions with Jupyter Notebook. This knowledge will form the foundation for all your Python programming.The course then merges these two powerful tools – Python and PostgreSQL. You will learn how to Build a database-driven application with PostgreSQL and Python, enabling you to create dynamic and interactive web applications.Lastly, you'll enter the world of APIs. Building an API with Python, Django, PostgreSQL, and the REST Framework will be the final step in your journey. This will allow your applications to communicate with other applications, providing powerful and flexible functionality.By the end of the course, you will have a thorough understanding of Python programming and PostgreSQL databases, and you will be able to create sophisticated web applications and APIs. Whether you're just starting your coding journey or looking to enhance your skills, this course will provide the knowledge and experience you need to succeed.
Overview
Section 1: PostgreSQL Setup
Lecture 1 Introduction
Lecture 2 What is PostgreSQL
Lecture 3 PostgreSQL Installation Requirements
Lecture 4 Install PostgreSQL for Windows
Lecture 5 Install PostgreSQL for Mac
Lecture 6 Installing PgAdmin on Mac
Lecture 7 Connecting PgAdmin to PostgreSQL
Lecture 8 Install Sample Database
Section 2: PostgreSQL Fundamentals
Lecture 9 Database Concepts
Lecture 10 PostgreSQL Data Types
Lecture 11 PostgreSQL Unique Constraint
Lecture 12 Filtering data using WHERE Clause
Lecture 13 Retrieving all data from a table
Lecture 14 Retrieving data from a specific column
Lecture 15 Removing duplicate records
Lecture 16 Sorting Data
Lecture 17 Grouping Data
Lecture 18 Using the HAVING Clause
Lecture 19 Truncating a table
Lecture 20 Stored Procedures
Section 3: Performing CRUD Operation with PostgreSQL
Lecture 21 What is CRUD
Lecture 22 Create database with pgadmin
Lecture 23 Create a table with pgadmin
Lecture 24 Create a table with SQL Statement
Lecture 25 Inserting records
Lecture 26 Retrieve data with SQL SELECT Statement
Lecture 27 Retrieving data with a subquery
Lecture 28 Updating existing records
Lecture 29 Deleting records
Section 4: PostgreSQL Table Joins
Lecture 30 Introduction to table joins
Lecture 31 INNER Table Join
Lecture 32 LEFT Table Join
Lecture 33 FULL OUTER Table Join
Lecture 34 NATURAL Table Join
Lecture 35 CROSS Table Join
Section 5: PostgreSQL Aggregate Functions
Lecture 36 Introduction to Aggregate Functions
Lecture 37 AVG Aggregate Functions
Lecture 38 COUNT Aggregate Functions
Lecture 39 MAX Aggregate Functions
Lecture 40 MIN Aggregate Functions
Lecture 41 SUM Aggregate Functions
Section 6: PostgreSQL Triggers
Lecture 42 Introduction to triggers
Lecture 43 Creating a trigger - Part 1
Lecture 44 Creating a trigger - Part 2
Lecture 45 Creating a trigger - Part 3
Lecture 46 Managing triggers
Section 7: Setting Up Python Programming Environment
Lecture 47 What is Python
Lecture 48 What is Jupyter Notebook
Lecture 49 Installing Jupyter Notebook Server
Lecture 50 Running Jupyter Notebook Server
Lecture 51 Notebook Dashboard
Lecture 52 Jupyter Notebook Interface
Lecture 53 Creating a new Notebook
Section 8: Python Programming Fundamentals
Lecture 54 Python Expressions
Lecture 55 Python Statements
Lecture 56 Python Comments
Lecture 57 Python Data Types
Lecture 58 Python Variables
Lecture 59 Python List
Lecture 60 Python Dictionaries
Lecture 61 Python Operators
Lecture 62 Python Conditional Statements
Lecture 63 Python Loops
Lecture 64 Python Functions
Section 9: Build a database driven app with PostgreSQL and Python
Lecture 65 What we will create
Lecture 66 Create a database and table
Lecture 67 Create sequence and alter table column
Lecture 68 Installing Python on Windows
Lecture 69 Installing Python on Macs
Lecture 70 Note on text editor
Lecture 71 Installing Text Editor
Lecture 72 Project design
Lecture 73 Create project directory and Python file
Lecture 74 Create the application interface - Part 1
Lecture 75 Create the application interface - Part 2
Lecture 76 Create and activate a virtual environment
Lecture 77 Install Python Database Connector
Lecture 78 Create database configuration file
Lecture 79 Connecting to PostgreSQL Database from Python file
Lecture 80 Create a class and methods
Lecture 81 Create standalone functions
Lecture 82 Binding button widgets to functions
Lecture 83 Testing the application
Lecture 84 Viewing database data with SQL
Lecture 85 Project Code
Section 10: Build API Using (Python , Django ,PostgreSQL,REST Framework)
Lecture 86 What is an API
Lecture 87 What is REST Framework
Lecture 88 What is Django
Lecture 89 Creating and activating a virtual environment
Lecture 90 Installing Django
Lecture 91 Installing REST Framework
Lecture 92 Installing Corsheaders
Lecture 93 Create a new Django Project
Lecture 94 Django App vs Django Project
Lecture 95 Registering Django Apps
Lecture 96 Django PostgreSQL Database Setup
Lecture 97 Applying initial migration
Lecture 98 Creating a serializer class
Lecture 99 Starting and stopping Django Server
Lecture 100 Creating a superuser account
Lecture 101 Creating views - Part 1
Lecture 102 Creating views - Part 2
Lecture 103 Mapping views to URLS
Lecture 104 Register model with Admin Site
Lecture 105 Creating model objects
Lecture 106 Installing Postman
Lecture 107 Testing API
Lecture 108 Project Code
Beginner Programmers: Individuals who are starting their journey in programming and want to learn Python and PostgreSQL.
This course provides foundational knowledge in Python and database management with PostgreSQL.,Experienced Programmers: Those already familiar with programming concepts but want to expand their knowledge into Python and PostgreSQL. This course can act as an efficient refresher or skill enhancer.,Database Administrators: Those who want to gain or enhance their understanding of PostgreSQL, including CRUD operations, table joins, triggers, and aggregate functions.,Web Developers: Those looking to build database-driven applications and APIs using Python, Django, and PostgreSQL. This course covers the use of Django REST Framework, which is widely used for API development.,Data Analysts and Data Scientists: Those who work with data regularly and want to enhance their data retrieval and manipulation skills using PostgreSQL. Python is also widely used in data analysis and machine learning, making this a good course for data professionals.,Career Changers: Individuals looking to shift their career into the tech industry, especially into roles such as software development, web development, or database administration.,IT Professionals: Those who wish to upskill by learning a modern programming language like Python and a robust database system like PostgreSQL.,Students: Those studying computer science or a related field, seeking to augment their academic knowledge with practical skills in Python and PostgreSQL.,This course is designed to cater to a broad spectrum of learners, ranging from
=========================================
*
*
==========================================